การเขียนโค้ด Android เพื่ออ่านบัตรประชาชน ผ่านเครื่องอ่านบัตรสมาร์ทการ์ด
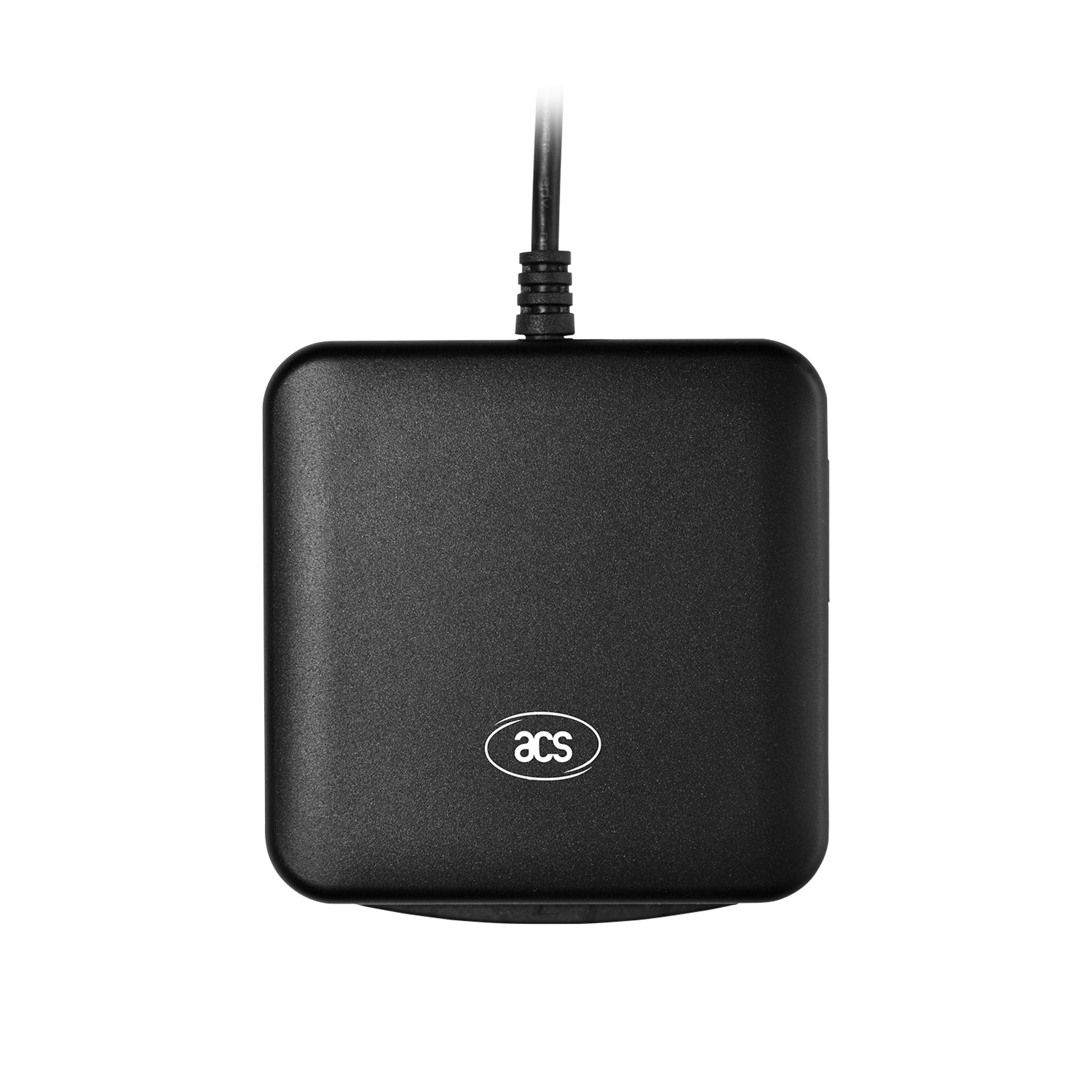
จำเป็นต้องใช้ Android USB Host API สำหรับการเชื่อมต่อกับเครื่องอ่านบัตรและ APDU Commands สำหรับการสื่อสารกับบัตรสมาร์ทการ์ด
ตัวอย่างโค้ด Android (Java)
- เพิ่ม Permissions ใน
AndroidManifest.xml
<uses-permission android:name="android.permission.USB_PERMISSION" />
<uses-feature android:name="android.hardware.usb.host" />
<application>
<meta-data
android:name="android.hardware.usb.action.USB_DEVICE_ATTACHED"
android:resource="@xml/device_filter" />
</application>
Code language: HTML, XML (xml)
- สร้างไฟล์
res/xml/device_filter.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<usb-device vendor-id="1839" product-id="8721" />
</resources>
Code language: HTML, XML (xml)
(ปรับ
vendor-id
และ
product-id
ให้ตรงกับเครื่องอ่านบัตรของคุณ)
- โค้ดสำหรับเชื่อมต่อและอ่านข้อมูลบัตร
import android.hardware.usb.*;
import android.content.Context;
public class SmartCardReader {
private UsbManager usbManager;
private UsbDevice device;
private UsbDeviceConnection connection;
private UsbEndpoint inEndpoint, outEndpoint;
public SmartCardReader(Context context) {
usbManager = (UsbManager) context.getSystemService(Context.USB_SERVICE);
}
public void connect(UsbDevice device) {
this.device = device;
// Request permission if needed
if (!usbManager.hasPermission(device)) {
// Implement permission dialog
return;
}
// Open connection to the device
UsbInterface usbInterface = device.getInterface(0);
connection = usbManager.openDevice(device);
connection.claimInterface(usbInterface, true);
for (int i = 0; i < usbInterface.getEndpointCount(); i++) {
UsbEndpoint endpoint = usbInterface.getEndpoint(i);
if (endpoint.getType() == UsbConstants.USB_ENDPOINT_XFER_BULK) {
if (endpoint.getDirection() == UsbConstants.USB_DIR_IN) {
inEndpoint = endpoint;
} else {
outEndpoint = endpoint;
}
}
}
}
public String sendApduCommand(byte[] command) {
if (connection == null || outEndpoint == null || inEndpoint == null) {
return "Device not connected";
}
// Send APDU command
connection.bulkTransfer(outEndpoint, command, command.length, 5000);
// Receive response
byte[] response = new byte[256];
int received = connection.bulkTransfer(inEndpoint, response, response.length, 5000);
if (received > 0) {
return bytesToHex(response, received);
}
return "No response";
}
private String bytesToHex(byte[] bytes, int length) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < length; i++) {
sb.append(String.format("%02X", bytes[i]));
}
return sb.toString();
}
}
Code language: PHP (php)
- APDU Command สำหรับบัตรประชาชนไทย
- Select File:
00 A4 00 00 02 11 11
- Read Data:
00 B0 00 00 20
(20 = จำนวนไบต์ที่ต้องการอ่าน)
การใช้งานใน Activity
UsbDevice device = ... // ค้นหา UsbDevice ผ่าน usbManager.getDeviceList()
SmartCardReader reader = new SmartCardReader(this);
reader.connect(device);
// ส่งคำสั่ง APDU เพื่อเลือกไฟล์
String response = reader.sendApduCommand(new byte[]{0x00, (byte) 0xA4, 0x00, 0x00, 0x02, 0x11, 0x11});
Log.d("APDU Response", response);
Code language: JavaScript (javascript)
หมายเหตุ
- ทดสอบการเชื่อมต่อผ่านเครื่องอ่านบัตร ACS ก่อนใช้งานจริง
- ใช้ APDU Commands ที่เหมาะสมกับรูปแบบข้อมูลในบัตร
- ตรวจสอบ
vendor-id
และproduct-id
ให้ตรงกับเครื่องอ่านของคุณ