การสร้างแชทบอทด้วย FastAPI และ Ollama API
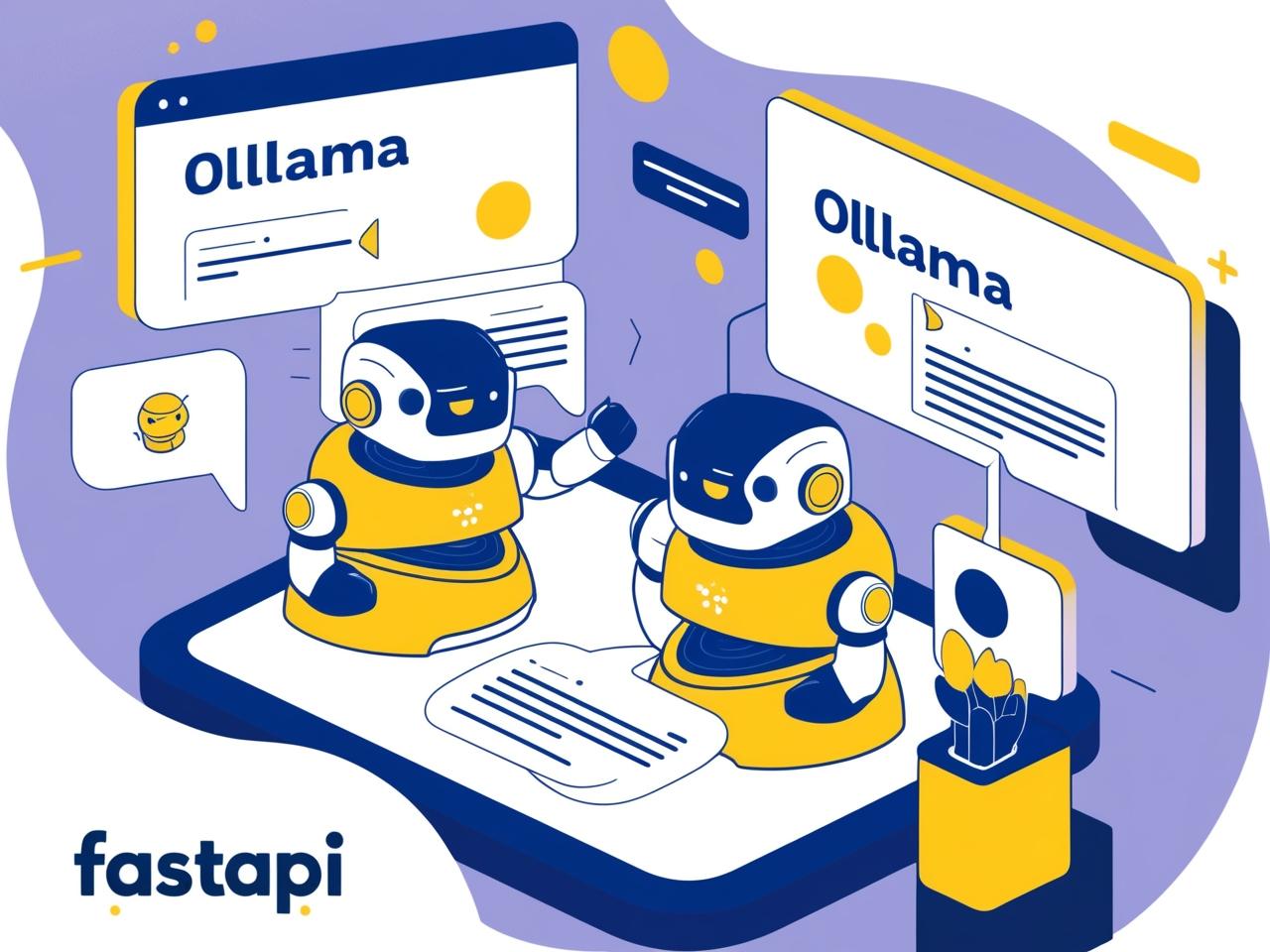
ในบทความนี้ เราจะอธิบายโค้ดที่ใช้สร้างแอปพลิเคชันแชทบอทโดยใช้ FastAPI และเชื่อมต่อกับ Ollama API ซึ่งเป็นโมเดลประมวลผลภาษาธรรมชาติ (NLP) ที่สามารถรันในเครื่องของเราเองได้ โดยโค้ดนี้ทำหน้าที่รับข้อความจากผู้ใช้ ส่งไปยัง Ollama API และแสดงผลลัพธ์กลับมาในรูปแบบเว็บอินเตอร์เฟซ
ส่วนประกอบของโค้ด
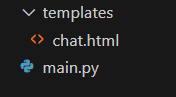
โค้ดนี้ใช้ไลบรารีดังต่อไปนี้:
- FastAPI: ใช้สำหรับสร้าง API
- Uvicorn: ใช้สำหรับรันเซิร์ฟเวอร์ FastAPI
- Requests: ใช้สำหรับส่งคำขอ HTTP ไปยัง Ollama API
- Pydantic: ใช้สำหรับตรวจสอบโครงสร้างของข้อมูลที่รับเข้ามา
สร้างไฟล์ main.py
1. กำหนดค่าพื้นฐาน
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
import requests
import uvicorn
from pydantic import BaseModel
from requests.exceptions import RequestException, JSONDecodeError
Code language: Python (python)
นำเข้าโมดูลที่จำเป็นสำหรับการสร้าง API และการจัดการคำขอ HTTP
2. กำหนดค่า API ของ Ollama
OLLAMA_API_URL = "http://localhost:11434/api/generate"
app = FastAPI()
Code language: Python (python)
-
OLLAMA_API_URL
กำหนด URL ของ Ollama API ที่รันอยู่ในเครื่องของเรา -
app = FastAPI()
สร้างอินสแตนซ์ของ FastAPI เพื่อกำหนดเส้นทาง API
3. ฟังก์ชันเรียกใช้โมเดล Ollama
def chat_with_ollama(prompt, model="llama3.2:latest"):
payload = {
"model": model,
"prompt": prompt,
"stream": False
}
headers = {"Content-Type": "application/json"}
try:
response = requests.post(OLLAMA_API_URL, json=payload, headers=headers)
response.raise_for_status()
try:
return response.json()["response"]
except JSONDecodeError:
return "Error: Invalid JSON response from Ollama API."
except RequestException as e:
return f"Error: {str(e)}"
Code language: Python (python)
- ฟังก์ชันนี้ใช้ส่งข้อความไปยัง Ollama API และรับคำตอบกลับ
- กำหนดค่า
model
ให้เป็น llama3.2:latest (สามารถเปลี่ยนเป็นเวอร์ชันอื่นได้) - ใช้
requests.post()
ส่งคำขอไปยัง API และจัดการข้อผิดพลาดที่อาจเกิดขึ้น
4. กำหนดโครงสร้างของข้อความแชท
class ChatMessage(BaseModel):
message: str
Code language: Python (python)
ใช้ Pydantic กำหนดโครงสร้างของข้อมูลที่รับจากผู้ใช้
5. สร้างหน้าเว็บแสดงแชท
@app.get("/", response_class=HTMLResponse)
async def get_chat_page():
try:
with open("templates/chat.html", "r", encoding="utf-8") as f:
html_content = f.read()
except UnicodeDecodeError:
with open("templates/chat.html", "r", encoding="latin-1") as f:
html_content = f.read()
except FileNotFoundError:
return HTMLResponse(content="Error: HTML template not found.", status_code=404)
return HTMLResponse(content=html_content)
Code language: JavaScript (javascript)
- ฟังก์ชันนี้ทำหน้าที่เปิดไฟล์ chat.html ที่เก็บ UI ของแชทบอท และแสดงผลออกมา
- ใช้ try-except เพื่อจัดการกรณีที่ไฟล์หายไปหรือมีปัญหาเกี่ยวกับการเข้ารหัส
- ตัวอย่างไฟล์ chat.html คลิ้ก
6. สร้าง API รับข้อความและส่งไปยัง Ollama
@app.post("/chat")
async def chat(message: ChatMessage):
user_message = message.message
if not user_message:
return {"response": "Error: Missing message."}
bot_response = chat_with_ollama(user_message)
return {"response": bot_response}
Code language: Python (python)
- รับข้อความจากผู้ใช้ผ่าน API
/chat
- ตรวจสอบว่าผู้ใช้ส่งข้อความมาหรือไม่
- ส่งข้อความไปยังฟังก์ชัน
chat_with_ollama
และคืนค่าผลลัพธ์
7. รันเซิร์ฟเวอร์
if __name__ == "__main__":
uvicorn.run(app, host="127.0.0.1", port=8001)
Code language: Python (python)
- ใช้ Uvicorn เพื่อรัน FastAPI บน
localhost:8001
วิธีการใช้งาน
- ติดตั้งไลบรารีที่จำเป็น: pip install fastapi uvicorn requests pydantic
- รัน Ollama API ก่อน: ollama serve
- รัน FastAPI: python main.py
- เปิดเบราว์เซอร์ไปที่
http://127.0.0.1:8001/
เพื่อใช้งานแชทบอท
โค้ดนี้เป็นตัวอย่างการสร้างแชทบอทโดยใช้ FastAPI และ Ollama API ซึ่งสามารถนำไปขยายต่อได้
เช่น:
- เพิ่มระบบจดจำประวัติการสนทนา
- ใช้ WebSocket สำหรับการสนทนาแบบเรียลไทม์
- ปรับแต่ง UI ของหน้าแชทให้ดูสวยงามขึ้น
หวังว่าบทความนี้จะช่วยให้เข้าใจการทำงานของระบบแชทบอทมากขึ้น!